Asynchronous Bayesian optimization with Trieste#
In this notebook we demonstrate Trieste’s ability to perform asynchronous Bayesian optimisation, as is suitable for scenarios where the objective function can be run for several points in parallel but where observations might return back at different times. To avoid wasting resources waiting for the evaluation of the whole batch, we immediately request the next point asynchronously, taking into account points that are still being evaluated. Besides saving resources, asynchronous approach also can potentially improve sample efficiency in comparison with synchronous batch strategies, although this is highly dependent on the use case.
To contrast this approach with regular batch optimization, this notebook also shows how to run parallel synchronous batch approach.
[1]:
# silence TF warnings and info messages, only print errors
# https://stackoverflow.com/questions/35911252/disable-tensorflow-debugging-information
import os
os.environ["TF_CPP_MIN_LOG_LEVEL"] = "3"
import tensorflow as tf
tf.get_logger().setLevel("ERROR")
import numpy as np
import time
import timeit
First, let’s define a simple objective that will emulate evaluations taking variable time. We will be using a classic Bayesian optimisation benchmark function Branin with a sleep call inserted in the middle of the calculation to emulate delay. Our sleep delay is a scaled sum of all input values to make sure delays are uneven.
[2]:
from trieste.objectives import ScaledBranin
def objective(points, sleep=True):
if points.shape[1] != 2:
raise ValueError(
f"Incorrect input shape, expected (*, 2), got {points.shape}"
)
observations = []
for point in points:
observation = ScaledBranin.objective(point)
if sleep:
# insert some artificial delay
# increases linearly with the absolute value of points
# which means our evaluations will take different time
delay = 3 * np.sum(point)
pid = os.getpid()
print(
f"Process {pid}: Objective: pretends like it's doing something for {delay:.2}s",
flush=True,
)
time.sleep(delay)
observations.append(observation)
return np.array(observations)
# test the defined objective function
objective(np.array([[0.1, 0.5]]), sleep=False)
[2]:
array([[-0.42052567]])
As always, we need to prepare the model and some initial data to kick-start the optimization process.
[3]:
from trieste.space import Box
from trieste.data import Dataset
search_space = Box([0, 0], [1, 1])
num_initial_points = 3
initial_query_points = search_space.sample(num_initial_points)
initial_observations = objective(initial_query_points.numpy(), sleep=False)
initial_data = Dataset(
query_points=initial_query_points,
observations=tf.constant(initial_observations, dtype=tf.float64),
)
import gpflow
from trieste.models.gpflow import GaussianProcessRegression, build_gpr
# We set the likelihood variance to a small number because
# we are dealing with a noise-free problem.
gpflow_model = build_gpr(initial_data, search_space, likelihood_variance=1e-7)
model = GaussianProcessRegression(gpflow_model)
# these imports will be used later for optimization
from trieste.acquisition import LocalPenalization
from trieste.acquisition.rule import (
AsynchronousGreedy,
EfficientGlobalOptimization,
)
from trieste.ask_tell_optimization import AskTellOptimizer
Multiprocessing setup#
To keep this notebook as reproducible as possible, we will only be using Python’s multiprocessing package here. In this section we will explain our setup and define some common code to be used later.
In both synchronous and asynchronous scenarios we will have a fixed set of worker processes performing observations. We will also have a main process responsible for optimization process with Trieste. When Trieste suggests a new point, it is inserted into a points queue. One of the workers picks this point from the queue, performs the observation, and inserts the output into the observations queue. The main process then picks up the observation from the queue, at which moment it either waits for the rest of the points in the batch to come back (synchronous scenario) or immediately suggests a new point (asynchronous scenario). This process continues either for a certain number of iterations or until we accumulate necessary number of observations.
The overall setup is illustrated in this diagram:
[4]:
# Necessary multiprocessing primitives
from multiprocessing import Manager, Process
We now define several common functions to implement the described setup. First we define a worker function that will be running a single observation in a separate process. Worker takes both queues as an input, reads next point from the points queue, makes an observation, and inserts observed data into the observations queue.
[5]:
def observer_proc(points_queue, observations_queue):
pid = os.getpid()
while True:
point_to_observe = points_queue.get()
if point_to_observe is None:
return
print(
f"Process {pid}: Observer : observing data at point {point_to_observe}",
flush=True,
)
new_observation = objective(point_to_observe, sleep=enable_sleep_delays)
new_data = (point_to_observe, new_observation)
print(f"Process {pid}: Observer : observed data {new_data}", flush=True)
observations_queue.put(new_data)
Next we define two helper functions, one is to create a certain number of worker processes, and another is to terminate them once we are done.
[6]:
def create_worker_processes(n_workers, points_queue, obseverations_queue):
observer_processes = []
for i in range(n_workers):
worker_proc = Process(
target=observer_proc, args=(points_queue, obseverations_queue)
)
worker_proc.daemon = True
worker_proc.start()
observer_processes.append(worker_proc)
return observer_processes
def terminate_processes(processes):
for prc in processes:
prc.terminate()
prc.join()
prc.close()
Finally we set some common parameters. See comments below for explanation of what each one means.
[7]:
# Number of worker processes to run simultaneously
# Setting this to 1 will turn both setups into non-batch sequential optimization
num_workers = 3
# Number of iterations to run the sycnhronous scenario for
num_iterations = 10
# Number of observations to collect in the asynchronous scenario
num_observations = num_workers * num_iterations
# Set this flag to False to disable sleep delays in case you want the notebook to execute quickly
enable_sleep_delays = True
Asynchronous optimization#
This section runs the asynchronous optimization routine. We first setup the ask/tell optimizer as we cannot hand over the evaluation of the objective to Trieste. Next we create thread-safe queues for points and observations, and run the optimization loop.
Crucially, even though we are using batch acquisition function Local Penalization, we specify batch size of 1. This is because we don’t really want a batch. Since the amount of workers we have is fixed, whenever we see a new observation we only need one point back. However this process can only be done with acquisition functions that implement greedy batch collection strategies, because they are able to take into account points that are currently being observed (in Trieste we call them “pending”). Trieste currently provides two such functions: Local Penalization and GIBBON. Notice that we use AsynchronousGreedy rule specifically designed for using greedy batch acquisition functions in asynchronous scenarios.
[8]:
# setup Ask Tell BO
local_penalization_acq = LocalPenalization(search_space, num_samples=2000)
local_penalization_rule = AsynchronousGreedy(builder=local_penalization_acq) # type: ignore
async_bo = AskTellOptimizer(
search_space, initial_data, model, local_penalization_rule
)
# retrieve process id for nice logging
pid = os.getpid()
# create point and observation queues
m = Manager()
pq = m.Queue()
oq = m.Queue()
# keep track of all workers we have launched
observer_processes = []
# counter to keep track of collected observations
points_observed = 0
start = timeit.default_timer()
try:
observer_processes = create_worker_processes(num_workers, pq, oq)
# init the queue with first batch of points
for _ in range(num_workers):
point = async_bo.ask()
pq.put(np.atleast_2d(point.numpy()))
while points_observed < num_observations:
# keep asking queue for new observations until one arrives
try:
new_data = oq.get_nowait()
print(
f"Process {pid}: Main : received data {new_data}",
flush=True,
)
except:
continue
# new_data is a tuple of (point, observation value)
# here we turn it into a Dataset and tell of it Trieste
points_observed += 1
new_data = Dataset(
query_points=tf.constant(new_data[0], dtype=tf.float64),
observations=tf.constant(new_data[1], dtype=tf.float64),
)
async_bo.tell(new_data)
# now we can ask Trieste for one more point
# and feed that back into the points queue
point = async_bo.ask()
print(f"Process {pid}: Main : acquired point {point}", flush=True)
pq.put(np.atleast_2d(point))
finally:
terminate_processes(observer_processes)
stop = timeit.default_timer()
# Collect the observations, compute the running time
async_lp_observations = (
async_bo.to_result().try_get_final_dataset().observations
- ScaledBranin.minimum
)
async_lp_time = stop - start
print(f"Got {len(async_lp_observations)} observations in {async_lp_time:.2f}s")
Process 3254: Observer : observing data at point [[0.2403252 0.27150085]]
Process 3254: Objective: pretends like it's doing something for 1.5s
Process 3254: Observer : observed data (array([[0.2403252 , 0.27150085]]), array([[-0.45770331]]))
Process 3258: Observer : observing data at point [[0.29152633 0.37654441]]
Process 3258: Objective: pretends like it's doing something for 2.0s
Process 3217: Main : received data (array([[0.2403252 , 0.27150085]]), array([[-0.45770331]]))
Process 3262: Observer : observing data at point [[0.18524113 0.35164288]]
Process 3262: Objective: pretends like it's doing something for 1.6s
Process 3262: Observer : observed data (array([[0.18524113, 0.35164288]]), array([[-0.51265757]]))
Process 3258: Observer : observed data (array([[0.29152633, 0.37654441]]), array([[-0.67512817]]))
Process 3217: Main : acquired point [[0.33347235 0.29365 ]]
Process 3254: Observer : observing data at point [[0.33347235 0.29365 ]]Process 3217: Main : received data (array([[0.18524113, 0.35164288]]), array([[-0.51265757]]))
Process 3254: Objective: pretends like it's doing something for 1.9s
Process 3217: Main : acquired point [[0.3715289 0.38210725]]
Process 3217: Main : received data (array([[0.29152633, 0.37654441]]), array([[-0.67512817]]))
Process 3262: Observer : observing data at point [[0.3715289 0.38210725]]
Process 3262: Objective: pretends like it's doing something for 2.3s
Process 3254: Observer : observed data (array([[0.33347235, 0.29365 ]]), array([[-0.6289444]]))
Process 3217: Main : acquired point [[0.43493402 0.31961732]]
Process 3217: Main : received data (array([[0.33347235, 0.29365 ]]), array([[-0.6289444]]))
Process 3258: Observer : observing data at point [[0.43493402 0.31961732]]
Process 3258: Objective: pretends like it's doing something for 2.3s
Process 3262: Observer : observed data (array([[0.3715289 , 0.38210725]]), array([[-0.70028757]]))
Process 3217: Main : acquired point [[0.37919769 0.35053482]]
Process 3217: Main : received data (array([[0.3715289 , 0.38210725]]), array([[-0.70028757]]))
Process 3254: Observer : observing data at point [[0.37919769 0.35053482]]
Process 3254: Objective: pretends like it's doing something for 2.2s
Process 3258: Observer : observed data (array([[0.43493402, 0.31961732]]), array([[-0.83763184]]))
Process 3217: Main : acquired point [[0.3679465 0.35314539]]
Process 3217: Main : received data (array([[0.43493402, 0.31961732]]), array([[-0.83763184]]))
Process 3262: Observer : observing data at point [[0.3679465 0.35314539]]
Process 3262: Objective: pretends like it's doing something for 2.2s
Process 3254: Observer : observed data (array([[0.37919769, 0.35053482]]), array([[-0.71812963]]))
Process 3217: Main : acquired point [[0.60307055 0.31548992]]
Process 3217: Main : received data (array([[0.37919769, 0.35053482]]), array([[-0.71812963]]))
Process 3258: Observer : observing data at point [[0.60307055 0.31548992]]
Process 3258: Objective: pretends like it's doing something for 2.8s
Process 3262: Observer : observed data (array([[0.3679465 , 0.35314539]]), array([[-0.70193284]]))
Process 3217: Main : acquired point [[0.51746937 0.25478461]]
Process 3217: Main : received data (array([[0.3679465 , 0.35314539]]), array([[-0.70193284]]))
Process 3254: Observer : observing data at point [[0.51746937 0.25478461]]
Process 3254: Objective: pretends like it's doing something for 2.3s
Process 3258: Observer : observed data (array([[0.60307055, 0.31548992]]), array([[-0.79691482]]))
Process 3217: Main : acquired point [[0.55104222 0.21455848]]
Process 3262: Observer : observing data at point [[0.55104222 0.21455848]]Process 3217: Main : received data (array([[0.60307055, 0.31548992]]), array([[-0.79691482]]))
Process 3262: Objective: pretends like it's doing something for 2.3s
Process 3254: Observer : observed data (array([[0.51746937, 0.25478461]]), array([[-1.00501472]]))
Process 3217: Main : acquired point [[0.51081444 0.29428914]]
Process 3217: Main : received data (array([[0.51746937, 0.25478461]]), array([[-1.00501472]]))
Process 3258: Observer : observing data at point [[0.51081444 0.29428914]]
Process 3258: Objective: pretends like it's doing something for 2.4s
Process 3262: Observer : observed data (array([[0.55104222, 0.21455848]]), array([[-1.02522882]]))
Process 3217: Main : acquired point [[0.5754338 0.12394976]]
Process 3254: Observer : observing data at point [[0.5754338 0.12394976]]Process 3217: Main : received data (array([[0.55104222, 0.21455848]]), array([[-1.02522882]]))
Process 3254: Objective: pretends like it's doing something for 2.1s
Process 3258: Observer : observed data (array([[0.51081444, 0.29428914]]), array([[-0.96856155]]))
Process 3217: Main : acquired point [[0.59417315 0. ]]
Process 3217: Main : received data (array([[0.51081444, 0.29428914]]), array([[-0.96856155]]))
Process 3262: Observer : observing data at point [[0.59417315 0. ]]
Process 3262: Objective: pretends like it's doing something for 1.8s
Process 3254: Observer : observed data (array([[0.5754338 , 0.12394976]]), array([[-1.02557192]]))
Process 3217: Main : acquired point [[0.55077419 0.09194282]]
Process 3217: Main : received data (array([[0.5754338 , 0.12394976]]), array([[-1.02557192]]))
Process 3258: Observer : observing data at point [[0.55077419 0.09194282]]
Process 3258: Objective: pretends like it's doing something for 1.9s
Process 3262: Observer : observed data (array([[0.59417315, 0. ]]), array([[-0.93613909]]))
Process 3217: Main : acquired point [[0.54302307 0.15781802]]
Process 3254: Observer : observing data at point [[0.54302307 0.15781802]]Process 3217: Main : received data (array([[0.59417315, 0. ]]), array([[-0.93613909]]))
Process 3254: Objective: pretends like it's doing something for 2.1s
Process 3258: Observer : observed data (array([[0.55077419, 0.09194282]]), array([[-1.03361749]]))
Process 3217: Main : acquired point [[0.5429238 0.16746332]]
Process 3217: Main : received data (array([[0.55077419, 0.09194282]]), array([[-1.03361749]]))
Process 3262: Observer : observing data at point [[0.5429238 0.16746332]]
Process 3262: Objective: pretends like it's doing something for 2.1s
Process 3254: Observer : observed data (array([[0.54302307, 0.15781802]]), array([[-1.04721815]]))
Process 3262: Observer : observed data (array([[0.5429238 , 0.16746332]]), array([[-1.0462965]]))
Process 3217: Main : acquired point [[0.54157247 0.15041018]]
Process 3217: Main : received data (array([[0.54302307, 0.15781802]]), array([[-1.04721815]]))
Process 3258: Observer : observing data at point [[0.54157247 0.15041018]]
Process 3258: Objective: pretends like it's doing something for 2.1s
Process 3217: Main : acquired point [[0. 0.84852557]]
Process 3217: Main : received data (array([[0.5429238 , 0.16746332]]), array([[-1.0462965]]))
Process 3254: Observer : observing data at point [[0. 0.84852557]]
Process 3254: Objective: pretends like it's doing something for 2.5s
Process 3258: Observer : observed data (array([[0.54157247, 0.15041018]]), array([[-1.04734306]]))
Process 3217: Main : acquired point [[1. 0.]]
Process 3262: Observer : observing data at point [[1. 0.]]Process 3217: Main : received data (array([[0.54157247, 0.15041018]]), array([[-1.04734306]]))
Process 3262: Objective: pretends like it's doing something for 3.0s
Process 3254: Observer : observed data (array([[0. , 0.84852557]]), array([[-0.42732077]]))
Process 3217: Main : acquired point [[0. 1.]]
Process 3217: Main : received data (array([[0. , 0.84852557]]), array([[-0.42732077]]))
Process 3258: Observer : observing data at point [[0. 1.]]
Process 3258: Objective: pretends like it's doing something for 3.0s
Process 3262: Observer : observed data (array([[1., 0.]]), array([[-0.84406373]]))
Process 3217: Main : acquired point [[0.54578821 0.14779043]]
Process 3217: Main : received data (array([[1., 0.]]), array([[-0.84406373]]))
Process 3254: Observer : observing data at point [[0.54578821 0.14779043]]
Process 3254: Objective: pretends like it's doing something for 2.1s
Process 3258: Observer : observed data (array([[0., 1.]]), array([[-0.71803081]]))
Process 3217: Main : acquired point [[0.84596823 0. ]]
Process 3217: Main : received data (array([[0., 1.]]), array([[-0.71803081]]))
Process 3262: Observer : observing data at point [[0.84596823 0. ]]
Process 3262: Objective: pretends like it's doing something for 2.5s
Process 3254: Observer : observed data (array([[0.54578821, 0.14779043]]), array([[-1.04719455]]))
Process 3217: Main : acquired point [[0.77301849 0. ]]
Process 3217: Main : received data (array([[0.54578821, 0.14779043]]), array([[-1.04719455]]))
Process 3258: Observer : observing data at point [[0.77301849 0. ]]
Process 3258: Objective: pretends like it's doing something for 2.3s
Process 3262: Observer : observed data (array([[0.84596823, 0. ]]), array([[-0.79455673]]))
Process 3217: Main : acquired point [[1. 0.20107373]]
Process 3254: Observer : observing data at point [[1. 0.20107373]]Process 3217: Main : received data (array([[0.84596823, 0. ]]), array([[-0.79455673]]))
Process 3254: Objective: pretends like it's doing something for 3.6s
Process 3258: Observer : observed data (array([[0.77301849, 0. ]]), array([[-0.66240019]]))
Process 3217: Main : acquired point [[0.48783993 0. ]]
Process 3217: Main : received data (array([[0.77301849, 0. ]]), array([[-0.66240019]]))
Process 3262: Observer : observing data at point [[0.48783993 0. ]]
Process 3262: Objective: pretends like it's doing something for 1.5s
Process 3262: Observer : observed data (array([[0.48783993, 0. ]]), array([[-0.8142595]]))
Process 3217: Main : acquired point [[0.92574896 0.12195717]]
Process 3258: Observer : observing data at point [[0.92574896 0.12195717]]Process 3217: Main : received data (array([[0.48783993, 0. ]]), array([[-0.8142595]]))
Process 3258: Objective: pretends like it's doing something for 3.1s
Process 3254: Observer : observed data (array([[1. , 0.20107373]]), array([[-1.01764555]]))
Process 3217: Main : acquired point [[0.1300595 1. ]]
Process 3217: Main : received data (array([[1. , 0.20107373]]), array([[-1.01764555]]))
Process 3262: Observer : observing data at point [[0.1300595 1. ]]
Process 3262: Objective: pretends like it's doing something for 3.4s
Process 3258: Observer : observed data (array([[0.92574896, 0.12195717]]), array([[-1.02022388]]))
Process 3217: Main : acquired point [[0.94533986 0.16029412]]
Process 3217: Main : received data (array([[0.92574896, 0.12195717]]), array([[-1.02022388]]))
Process 3254: Observer : observing data at point [[0.94533986 0.16029412]]
Process 3254: Objective: pretends like it's doing something for 3.3s
Process 3262: Observer : observed data (array([[0.1300595, 1. ]]), array([[-0.87952306]]))
Process 3217: Main : acquired point [[0.97285897 0.14256176]]
Process 3217: Main : received data (array([[0.1300595, 1. ]]), array([[-0.87952306]]))
Process 3258: Observer : observing data at point [[0.97285897 0.14256176]]
Process 3258: Objective: pretends like it's doing something for 3.3s
Process 3254: Observer : observed data (array([[0.94533986, 0.16029412]]), array([[-1.04157287]]))
Process 3217: Main : acquired point [[0.96846217 0.15092859]]
Process 3217: Main : received data (array([[0.94533986, 0.16029412]]), array([[-1.04157287]]))
Process 3262: Observer : observing data at point [[0.96846217 0.15092859]]
Process 3262: Objective: pretends like it's doing something for 3.4s
Process 3258: Observer : observed data (array([[0.97285897, 0.14256176]]), array([[-1.04031581]]))
Process 3217: Main : acquired point [[0.96556464 0.144619 ]]
Process 3217: Main : received data (array([[0.97285897, 0.14256176]]), array([[-1.04031581]]))
Process 3254: Observer : observing data at point [[0.96556464 0.144619 ]]
Process 3254: Objective: pretends like it's doing something for 3.3s
Process 3262: Observer : observed data (array([[0.96846217, 0.15092859]]), array([[-1.04471413]]))
Process 3217: Main : acquired point [[0.96469057 0.1701573 ]]
Process 3258: Observer : observing data at point [[0.96469057 0.1701573 ]]
Process 3258: Objective: pretends like it's doing something for 3.4sGot 33 observations in 54.78s
Synchronous parallel optimization#
This section runs the synchronous parallel optimization with Trieste. We again use Local Penalization acquisition function, but this time with batch size equal to the number of workers we have available. Once Trieste suggests the batch, we add all points to the point queue, and workers immediatelly pick them up, one point per worker. Therefore all points in the batch are evaluated in parallel.
[9]:
# setup Ask Tell BO
gpflow_model = build_gpr(initial_data, search_space, likelihood_variance=1e-7)
model = GaussianProcessRegression(gpflow_model)
local_penalization_acq = LocalPenalization(search_space, num_samples=2000)
local_penalization_rule = EfficientGlobalOptimization( # type: ignore
num_query_points=num_workers, builder=local_penalization_acq
)
sync_bo = AskTellOptimizer(
search_space, initial_data, model, local_penalization_rule
)
# retrieve process id for nice logging
pid = os.getpid()
# create point and observation queues
m = Manager()
pq = m.Queue()
oq = m.Queue()
# keep track of all workers we have launched
observer_processes = []
start = timeit.default_timer()
try:
observer_processes = create_worker_processes(num_workers, pq, oq)
# BO loop starts here
for i in range(num_iterations):
print(f"Process {pid}: Main : iteration {i} starts", flush=True)
# get a batch of points from Trieste, send them to points queue
# each worker picks up a point and processes it
points = sync_bo.ask()
for point in points.numpy():
pq.put(point.reshape(1, -1)) # reshape is to make point a 2d array
# now we wait for all workers to finish
# we create an empty dataset and wait
# until we collected as many observations in it
# as there were points in the batch
all_new_data = Dataset(
tf.zeros((0, initial_data.query_points.shape[1]), tf.float64),
tf.zeros((0, initial_data.observations.shape[1]), tf.float64),
)
while len(all_new_data) < num_workers:
# this line blocks the process until new data is available in the queue
new_data = oq.get()
print(
f"Process {pid}: Main : received data {new_data}",
flush=True,
)
new_data = Dataset(
query_points=tf.constant(new_data[0], dtype=tf.float64),
observations=tf.constant(new_data[1], dtype=tf.float64),
)
all_new_data = all_new_data + new_data
# tell Trieste of new batch of observations
sync_bo.tell(all_new_data)
finally:
terminate_processes(observer_processes)
stop = timeit.default_timer()
# Collect the observations, compute the running time
sync_lp_observations = (
sync_bo.to_result().try_get_final_dataset().observations
- ScaledBranin.minimum
)
sync_lp_time = stop - start
print(f"Got {len(sync_lp_observations)} observations in {sync_lp_time:.2f}s")
Process 3217: Main : iteration 0 starts
Process 3696: Observer : observing data at point [[0.18531786 0.35201863]]Process 3692: Observer : observing data at point [[0.29182154 0.37642715]]
Process 3688: Observer : observing data at point [[0.24009463 0.27160768]]Process 3692: Objective: pretends like it's doing something for 2.0s
Process 3688: Objective: pretends like it's doing something for 1.5sProcess 3696: Objective: pretends like it's doing something for 1.6s
Process 3688: Observer : observed data (array([[0.24009463, 0.27160768]]), array([[-0.45746013]]))
Process 3217: Main : received data (array([[0.24009463, 0.27160768]]), array([[-0.45746013]]))
Process 3696: Observer : observed data (array([[0.18531786, 0.35201863]]), array([[-0.51401977]]))
Process 3217: Main : received data (array([[0.18531786, 0.35201863]]), array([[-0.51401977]]))
Process 3692: Observer : observed data (array([[0.29182154, 0.37642715]]), array([[-0.6749724]]))
Process 3217: Main : received data (array([[0.29182154, 0.37642715]]), array([[-0.6749724]]))
Process 3217: Main : iteration 1 starts
Process 3692: Observer : observing data at point [[0.45669714 0.28498851]]Process 3688: Observer : observing data at point [[0.38640504 0.35040383]]Process 3696: Observer : observing data at point [[0.25633118 0.45024468]]
Process 3688: Objective: pretends like it's doing something for 2.2sProcess 3692: Objective: pretends like it's doing something for 2.2s
Process 3696: Objective: pretends like it's doing something for 2.1s
Process 3696: Observer : observed data (array([[0.25633118, 0.45024468]]), array([[-0.75749469]]))
Process 3217: Main : received data (array([[0.25633118, 0.45024468]]), array([[-0.75749469]]))
Process 3688: Observer : observed data (array([[0.38640504, 0.35040383]]), array([[-0.72951918]]))
Process 3217: Main : received data (array([[0.38640504, 0.35040383]]), array([[-0.72951918]]))
Process 3692: Observer : observed data (array([[0.45669714, 0.28498851]]), array([[-0.90194136]]))
Process 3217: Main : received data (array([[0.45669714, 0.28498851]]), array([[-0.90194136]]))
Process 3217: Main : iteration 2 starts
Process 3692: Observer : observing data at point [[0.48845918 0.20436758]]Process 3696: Observer : observing data at point [[0.56276928 0.21304923]]
Process 3688: Observer : observing data at point [[0.64579506 0.19825759]]
Process 3696: Objective: pretends like it's doing something for 2.3sProcess 3692: Objective: pretends like it's doing something for 2.1s
Process 3688: Objective: pretends like it's doing something for 2.5s
Process 3692: Observer : observed data (array([[0.48845918, 0.20436758]]), array([[-0.9892787]]))
Process 3217: Main : received data (array([[0.48845918, 0.20436758]]), array([[-0.9892787]]))
Process 3696: Observer : observed data (array([[0.56276928, 0.21304923]]), array([[-1.01399188]]))
Process 3217: Main : received data (array([[0.56276928, 0.21304923]]), array([[-1.01399188]]))
Process 3688: Observer : observed data (array([[0.64579506, 0.19825759]]), array([[-0.81826432]]))
Process 3217: Main : received data (array([[0.64579506, 0.19825759]]), array([[-0.81826432]]))
Process 3217: Main : iteration 3 starts
Process 3692: Observer : observing data at point [[0.53296942 0.22480206]]Process 3696: Observer : observing data at point [[0.53330867 0.23238647]]
Process 3696: Objective: pretends like it's doing something for 2.3s
Process 3688: Observer : observing data at point [[0.53474806 0.2175955 ]]
Process 3692: Objective: pretends like it's doing something for 2.3s
Process 3688: Objective: pretends like it's doing something for 2.3s
Process 3688: Observer : observed data (array([[0.53474806, 0.2175955 ]]), array([[-1.03069967]]))
Process 3217: Main : received data (array([[0.53474806, 0.2175955 ]]), array([[-1.03069967]]))
Process 3692: Observer : observed data (array([[0.53296942, 0.22480206]]), array([[-1.02692872]]))
Process 3217: Main : received data (array([[0.53296942, 0.22480206]]), array([[-1.02692872]]))
Process 3696: Observer : observed data (array([[0.53330867, 0.23238647]]), array([[-1.02234936]]))
Process 3217: Main : received data (array([[0.53330867, 0.23238647]]), array([[-1.02234936]]))
Process 3217: Main : iteration 4 starts
Process 3688: Observer : observing data at point [[0.57006975 0. ]]Process 3692: Observer : observing data at point [[0.01382251 0.66553788]]Process 3696: Observer : observing data at point [[0.55040352 0.11190446]]
Process 3692: Objective: pretends like it's doing something for 2.0s
Process 3688: Objective: pretends like it's doing something for 1.7sProcess 3696: Objective: pretends like it's doing something for 2.0s
Process 3688: Observer : observed data (array([[0.57006975, 0. ]]), array([[-0.95685287]]))
Process 3217: Main : received data (array([[0.57006975, 0. ]]), array([[-0.95685287]]))
Process 3696: Observer : observed data (array([[0.55040352, 0.11190446]]), array([[-1.04120001]]))
Process 3217: Main : received data (array([[0.55040352, 0.11190446]]), array([[-1.04120001]]))
Process 3692: Observer : observed data (array([[0.01382251, 0.66553788]]), array([[-0.00618616]]))
Process 3217: Main : received data (array([[0.01382251, 0.66553788]]), array([[-0.00618616]]))
Process 3217: Main : iteration 5 starts
Process 3692: Observer : observing data at point [[0.54428597 0.14813332]]Process 3688: Observer : observing data at point [[0.54372084 0.15108506]]Process 3696: Observer : observing data at point [[0.54315406 0.15404058]]
Process 3692: Objective: pretends like it's doing something for 2.1sProcess 3688: Objective: pretends like it's doing something for 2.1s
Process 3696: Objective: pretends like it's doing something for 2.1s
Process 3692: Observer : observed data (array([[0.54428597, 0.14813332]]), array([[-1.04732221]]))Process 3688: Observer : observed data (array([[0.54372084, 0.15108506]]), array([[-1.0473751]]))
Process 3217: Main : received data (array([[0.54428597, 0.14813332]]), array([[-1.04732221]]))
Process 3217: Main : received data (array([[0.54372084, 0.15108506]]), array([[-1.0473751]]))
Process 3696: Observer : observed data (array([[0.54315406, 0.15404058]]), array([[-1.04735997]]))
Process 3217: Main : received data (array([[0.54315406, 0.15404058]]), array([[-1.04735997]]))
Process 3217: Main : iteration 6 starts
Process 3692: Observer : observing data at point [[1. 0.]]Process 3696: Observer : observing data at point [[0.53302204 0.13538259]]
Process 3688: Observer : observing data at point [[0.53220238 0.13072826]]
Process 3692: Objective: pretends like it's doing something for 3.0s
Process 3688: Objective: pretends like it's doing something for 2.0sProcess 3696: Objective: pretends like it's doing something for 2.0s
Process 3688: Observer : observed data (array([[0.53220238, 0.13072826]]), array([[-1.04133216]]))
Process 3217: Main : received data (array([[0.53220238, 0.13072826]]), array([[-1.04133216]]))
Process 3696: Observer : observed data (array([[0.53302204, 0.13538259]]), array([[-1.04291056]]))
Process 3217: Main : received data (array([[0.53302204, 0.13538259]]), array([[-1.04291056]]))
Process 3692: Observer : observed data (array([[1., 0.]]), array([[-0.84406373]]))
Process 3217: Main : received data (array([[1., 0.]]), array([[-0.84406373]]))
Process 3217: Main : iteration 7 starts
Process 3688: Observer : observing data at point [[0.84595781 0. ]]Process 3696: Observer : observing data at point [[0.76194672 0. ]]
Process 3696: Objective: pretends like it's doing something for 2.3sProcess 3692: Observer : observing data at point [[0.90463932 0. ]]
Process 3692: Objective: pretends like it's doing something for 2.7s
Process 3688: Objective: pretends like it's doing something for 2.5s
Process 3696: Observer : observed data (array([[0.76194672, 0. ]]), array([[-0.65608714]]))
Process 3217: Main : received data (array([[0.76194672, 0. ]]), array([[-0.65608714]]))
Process 3688: Observer : observed data (array([[0.84595781, 0. ]]), array([[-0.79453156]]))
Process 3217: Main : received data (array([[0.84595781, 0. ]]), array([[-0.79453156]]))
Process 3692: Observer : observed data (array([[0.90463932, 0. ]]), array([[-0.91807916]]))
Process 3217: Main : received data (array([[0.90463932, 0. ]]), array([[-0.91807916]]))
Process 3217: Main : iteration 8 starts
Process 3696: Observer : observing data at point [[0.95104169 0.15640998]]Process 3692: Observer : observing data at point [[0.93408211 0.08602846]]Process 3688: Observer : observing data at point [[1. 0.2598046]]
Process 3688: Objective: pretends like it's doing something for 3.8s
Process 3696: Objective: pretends like it's doing something for 3.3sProcess 3692: Objective: pretends like it's doing something for 3.1s
Process 3692: Observer : observed data (array([[0.93408211, 0.08602846]]), array([[-1.01764763]]))
Process 3217: Main : received data (array([[0.93408211, 0.08602846]]), array([[-1.01764763]]))
Process 3696: Observer : observed data (array([[0.95104169, 0.15640998]]), array([[-1.04505786]]))
Process 3217: Main : received data (array([[0.95104169, 0.15640998]]), array([[-1.04505786]]))
Process 3688: Observer : observed data (array([[1. , 0.2598046]]), array([[-1.0022603]]))
Process 3217: Main : received data (array([[1. , 0.2598046]]), array([[-1.0022603]]))
Process 3217: Main : iteration 9 starts
Process 3692: Observer : observing data at point [[0.98507518 0.1664095 ]]Process 3688: Observer : observing data at point [[0.99128409 0.17634602]]
Process 3696: Observer : observing data at point [[0.98020332 0.15588816]]
Process 3688: Objective: pretends like it's doing something for 3.5sProcess 3692: Objective: pretends like it's doing something for 3.5s
Process 3696: Objective: pretends like it's doing something for 3.4s
Process 3696: Observer : observed data (array([[0.98020332, 0.15588816]]), array([[-1.03748338]]))
Process 3217: Main : received data (array([[0.98020332, 0.15588816]]), array([[-1.03748338]]))
Process 3692: Observer : observed data (array([[0.98507518, 0.1664095 ]]), array([[-1.0344702]]))
Process 3217: Main : received data (array([[0.98507518, 0.1664095 ]]), array([[-1.0344702]]))
Process 3688: Observer : observed data (array([[0.99128409, 0.17634602]]), array([[-1.02841351]]))
Process 3217: Main : received data (array([[0.99128409, 0.17634602]]), array([[-1.02841351]]))
Got 33 observations in 48.80s
Comparison#
To compare outcomes of sync and async runs, let’s plot their respective regrets side by side, and print out the running time. For this toy problem we expect async scenario to run a little bit faster on machines with multiple CPU.
[10]:
from trieste.experimental.plotting import plot_regret
import matplotlib.pyplot as plt
fig, ax = plt.subplots(1, 2)
sync_lp_min_idx = tf.squeeze(tf.argmin(sync_lp_observations, axis=0))
async_lp_min_idx = tf.squeeze(tf.argmin(async_lp_observations, axis=0))
plot_regret(
sync_lp_observations.numpy(),
ax[0],
num_init=len(initial_data),
idx_best=sync_lp_min_idx,
)
ax[0].set_yscale("log")
ax[0].set_ylabel("Regret")
ax[0].set_ylim(0.0000001, 100)
ax[0].set_xlabel("# evaluations")
ax[0].set_title(
f"Sync LP, {len(sync_lp_observations)} points, time {sync_lp_time:.2f}"
)
plot_regret(
async_lp_observations.numpy(),
ax[1],
num_init=len(initial_data),
idx_best=async_lp_min_idx,
)
ax[1].set_yscale("log")
ax[1].set_ylabel("Regret")
ax[1].set_ylim(0.0000001, 100)
ax[1].set_xlabel("# evaluations")
ax[1].set_title(
f"Async LP, {len(async_lp_observations)} points, time {async_lp_time:.2f}s"
)
fig.tight_layout()
/opt/hostedtoolcache/Python/3.10.12/x64/lib/python3.10/site-packages/matplotlib/_mathtext.py:1880: UserWarning: warn_name_set_on_empty_Forward: setting results name 'sym' on Forward expression that has no contained expression
- p.placeable("sym"))
/opt/hostedtoolcache/Python/3.10.12/x64/lib/python3.10/site-packages/matplotlib/_mathtext.py:1885: UserWarning: warn_ungrouped_named_tokens_in_collection: setting results name 'name' on ZeroOrMore expression collides with 'name' on contained expression
"{" + ZeroOrMore(p.simple | p.unknown_symbol)("name") + "}")
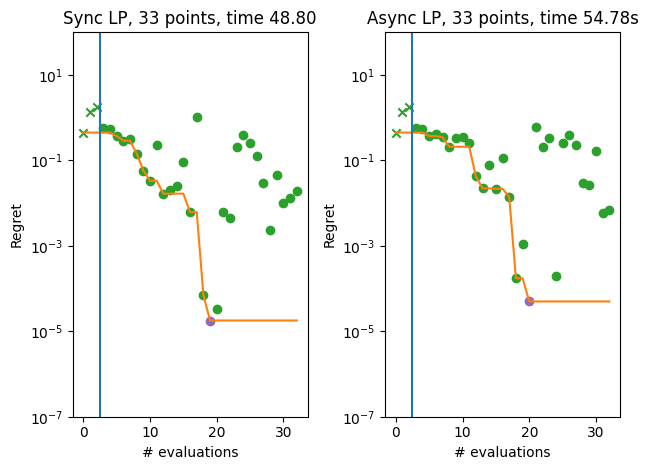